Introduction to SQL
In a world where data drives decisions, SQL (Structured Query Language) is the key to unlocking insights from databases. From healthcare systems tracking patient records to e-commerce giants analyzing sales trends, SQL powers industries globally. This guide will walk you through foundational concepts, enriched with real-world query examples, to help you build, manage, and analyze databases like a pro. Let’s get started!
1. What is SQL?
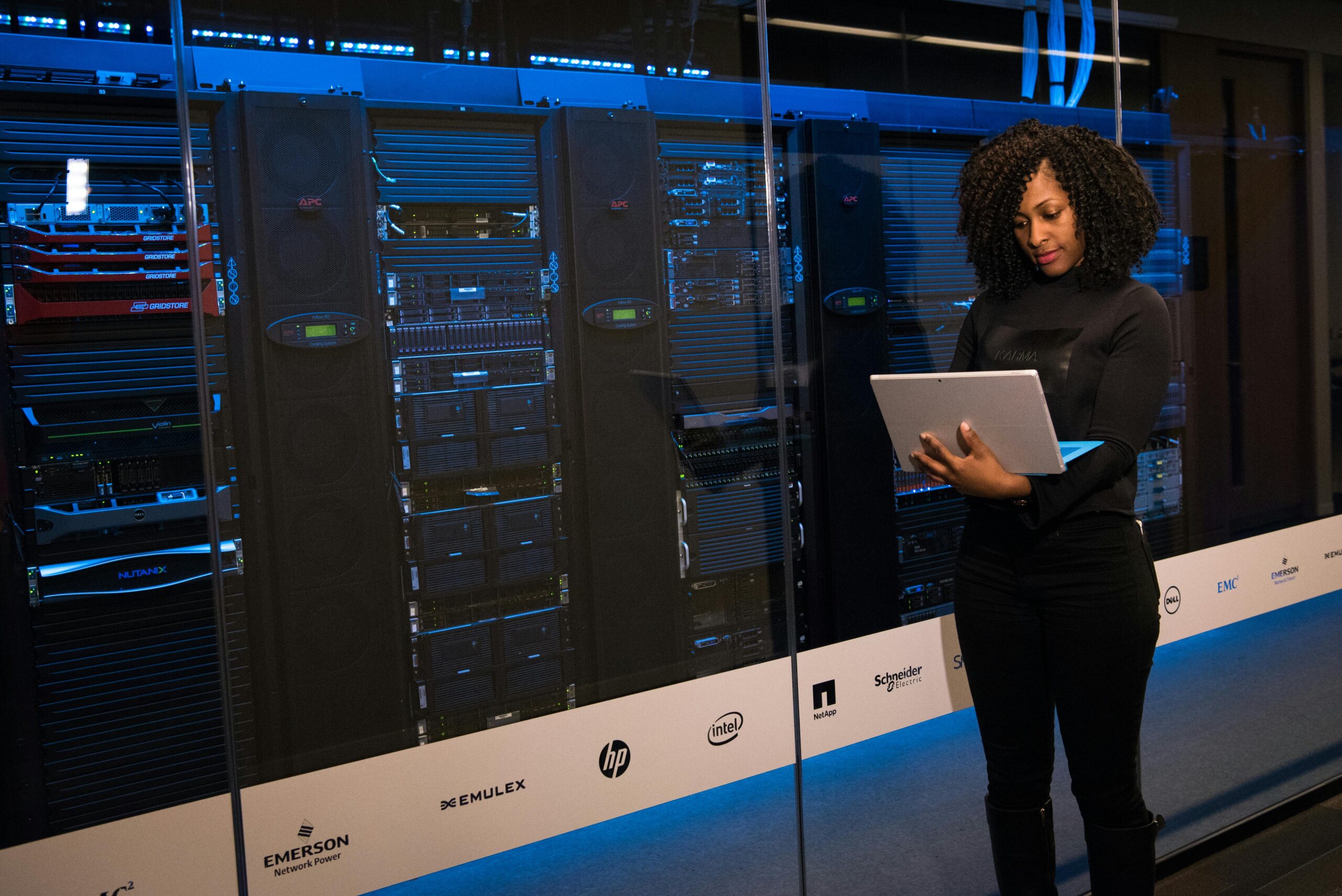
SQL is a domain-specific language for managing relational databases (data organized into tables with rows and columns). Developed in the 1970s by IBM, it’s now the standard for interacting with databases like MySQL, PostgreSQL, and Microsoft SQL Server.
Key Capabilities:
- CRUD Operations: Create, Read, Update, Delete data.
- Database Design: Define tables, relationships, and constraints.
- Advanced Analytics: Aggregate, filter, and join datasets.
Example Use Case:
A hospital database uses SQL to:
- Create patient records.
- Retrieve appointment schedules.
- Update treatment plans.
- Delete outdated entries.
2. Databases and Tables: The Building Blocks
A database is a structured collection of data. Imagine an online store’s database with tables like Products
, Customers
, and Orders
.
Sample Table: Products
product_id | product_name | price | category |
---|---|---|---|
101 | Laptop | 899 | Electronics |
102 | Desk Chair | 199 | Furniture |
Creating a Table:
CREATE TABLE Products ( product_id INT PRIMARY KEY, product_name VARCHAR(100) NOT NULL, price DECIMAL(10,2), category VARCHAR(50) );
3. Basic SQL Queries: Hands-On Examples
SELECT: Retrieve Data
Fetch all columns from the Products
table:
SELECT * FROM Products;
Filter products under $200 in the Furniture category:
SELECT product_name, price FROM Products WHERE category = 'Furniture' AND price < 200;
INSERT: Add New Data
Add a new product:
INSERT INTO Products (product_id, product_name, price, category) VALUES (103, 'Wireless Mouse', 29.99, 'Electronics');
UPDATE: Modify Existing Data
Increase the price of Laptops by 10%:
UPDATE Products SET price = price * 1.10 WHERE category = 'Electronics';
DELETE: Remove Data
Delete all products with a price below $20:
DELETE FROM Products WHERE price < 20;
4. SQL Clauses: Filter, Sort, and Limit
WHERE Clause
- Filter using
BETWEEN
:sqlCopySELECT * FROM Orders WHERE order_date BETWEEN ‘2023-01-01’ AND ‘2023-12-31’; - Match patterns with
LIKE
:sqlCopySELECT * FROM Customers WHERE email LIKE ‘%@gmail.com’;
ORDER BY
Sort customers by name (A-Z) and then by registration date (newest first):
SELECT name, email, registration_date FROM Customers ORDER BY name ASC, registration_date DESC;
LIMIT and OFFSET
Fetch the second-highest priced product:
SELECT product_name, price FROM Products ORDER BY price DESC LIMIT 1 OFFSET 1;
5. Data Types and Constraints
Common Data Types:
INT
: Whole numbers (e.g.,quantity
).VARCHAR(255)
: Variable-length text (e.g.,address
).DATE
: Dates (e.g.,birthdate
).BOOLEAN
: True/False values (e.g.,is_active
).
Key Constraints:
PRIMARY KEY
: Uniquely identifies a row.FOREIGN KEY
: Links to another table’s primary key.CHECK
: Ensures values meet a condition.
Example Table with Constraints:
CREATE TABLE Orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE NOT NULL, total_amount DECIMAL(10,2) CHECK (total_amount >= 0), FOREIGN KEY (customer_id) REFERENCES Customers(customer_id) );
6. SQL Joins: Combine Data from Multiple Tables
INNER JOIN
Fetch orders with customer details:
SELECT Orders.order_id, Customers.name, Orders.total_amount FROM Orders INNER JOIN Customers ON Orders.customer_id = Customers.customer_id;
LEFT JOIN
List all customers, including those without orders:
SELECT Customers.name, Orders.order_id FROM Customers LEFT JOIN Orders ON Customers.customer_id = Orders.customer_id;
FULL OUTER JOIN
Get all customers and orders, even if unmatched:
SELECT Customers.name, Orders.order_id FROM Customers FULL OUTER JOIN Orders ON Customers.customer_id = Orders.customer_id;
7. Aggregation Functions and GROUP BY
Calculate total sales by category:
SELECT category, SUM(price) AS total_sales FROM Products GROUP BY category;
Find categories with average price over $300:
SELECT category, AVG(price) AS avg_price FROM Products GROUP BY category HAVING AVG(price) > 300;
8. Indexes and Query Optimization
Create an Index for faster searches on customer_email
:
CREATE INDEX idx_email ON Customers(email);
Optimization Tips:
- Use
EXPLAIN
to analyze query execution plans:sqlCopyEXPLAIN SELECT * FROM Customers WHERE name = ‘John Doe’; - Avoid
SELECT *
; specify columns instead. - Break complex queries into subqueries.
9. SQL vs. NoSQL: When to Use Which?
Feature | SQL | NoSQL |
---|---|---|
Data Structure | Tables with fixed schemas | Flexible (JSON, documents) |
Scalability | Vertical scaling | Horizontal scaling |
Use Cases | Financial systems, ERP | Real-time apps (IoT, social media) |
10. Best Practices for Writing Clean SQL
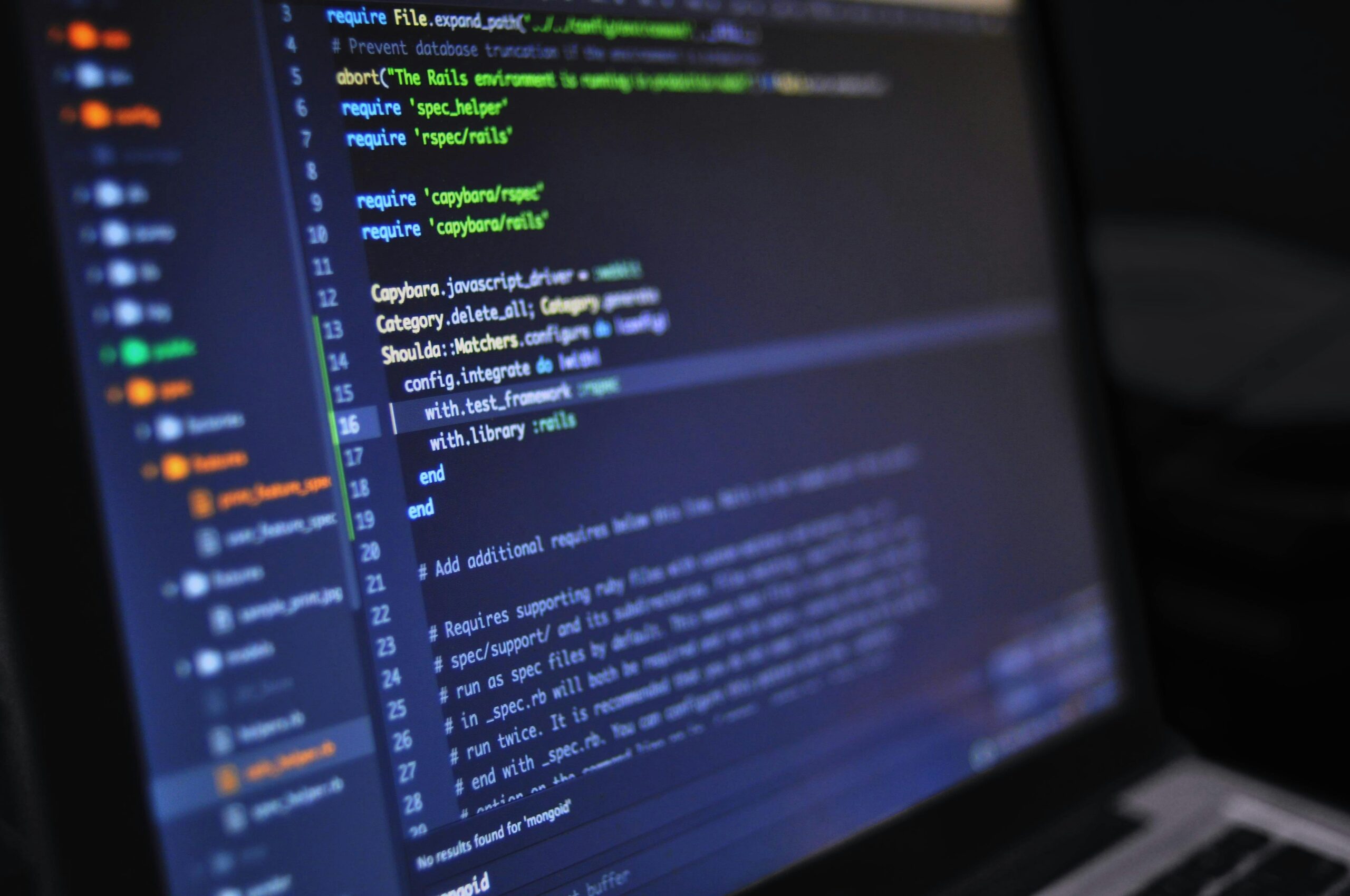
- Use Aliases for Readability:sqlCopySELECT p.product_name, c.name AS customer_name FROM Products p INNER JOIN Orders o ON p.product_id = o.product_id INNER JOIN Customers c ON o.customer_id = c.customer_id;
- Format Queries Consistently: Indent clauses like
WHERE
andJOIN
. - Backup Data Regularly: Use
mysqldump
or similar tools. - Sanitize Inputs to prevent SQL injection attacks.
Conclusion
Mastering SQL opens doors to careers in data analysis, software development, and beyond. Start by practicing the examples above using free tools like SQLite or MySQL Workbench. As you grow confident, explore window functions, stored procedures, and triggers. Remember, consistency is key—build a small project like a library management system or sales tracker to reinforce your skills! click here to read more blogs like this.
FAQs
1. How do I handle NULL values in SQL?
Use IS NULL
or IS NOT NULL
to filter:
SELECT * FROM Customers WHERE phone_number IS NULL;
2. What’s the difference between SQL and MySQL?
SQL is the language; MySQL is a database system that uses SQL.
3. Can I undo a DELETE operation?
Only if you use transactions. Wrap queries in BEGIN TRANSACTION
and COMMIT
or ROLLBACK
.
4. How do I export query results to a file?
In MySQL:
SELECT * FROM Products INTO OUTFILE '/tmp/products.csv' FIELDS TERMINATED BY ',';
5. What’s a subquery?
A query nested inside another query:
SELECT name FROM Customers WHERE customer_id IN ( SELECT customer_id FROM Orders WHERE total_amount > 500 );